Introduction
Push notification is the delivery of information to the user without a specific request from the user. In mobile devices, push notifications are implemented employing the use of a notification service. The notification is sent to each device that has registered to receive them, and is handled by the platform before giving it to the specific app. To implement push notification:
- App has to register itself (the device) to the platform specific notification service.
- Push messages can be sent via the notification service only, hence the backend/server code that handles it must connect to the notification service.
Push notifications can be broadcast, or specific.
Basic Architecture of Push Notifications
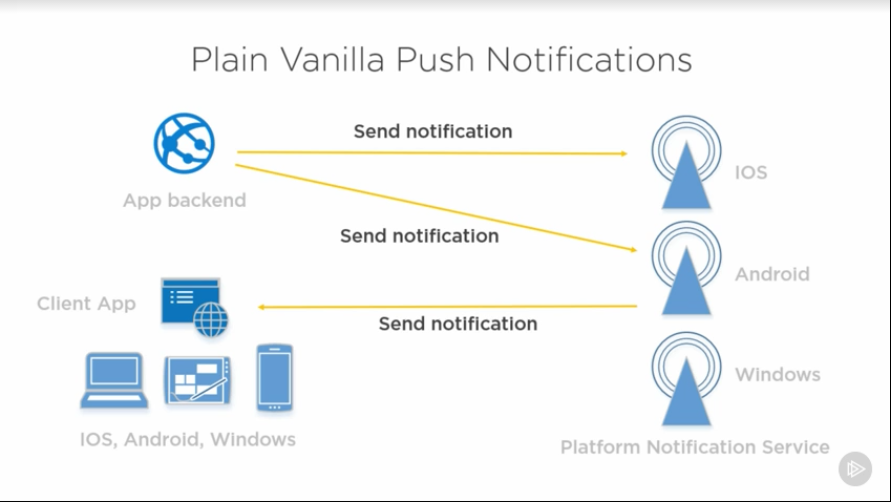
As observed, push notifications with the client app and the server can be routed only via the Push Notification Service (PNS) provided by each platform [APNS - Apple Push Notification Service, FCM - Firebase Cloud Messaging (which replaced Google Cloud Messaging in Sept 2016), etc]. Implementing connection between them are cumbersome, and this is where Azure Notification Hub comes into play.
Architecture of Push Notifications with Azure
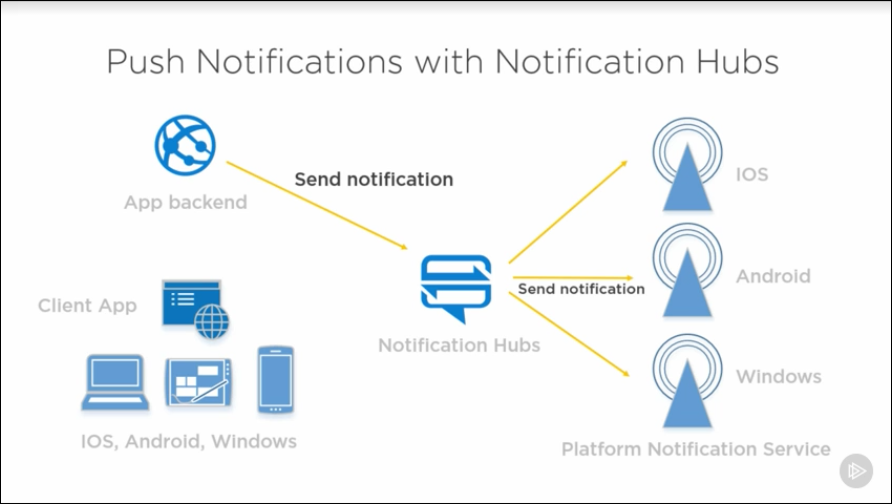
Azure provides a notification hub that talks to each PNS, giving the developer only one service to talk to (ANH). The basic architecture remains as such, but instead of the server talking to each required PNS, it needs to talk only with ANH. This greatly reduces overhead.
How to, the steps at a glance:
- Create a Mobile App in Azure
- Add Notification Hub to Mobile App
- Register in PNS
- Configure backend to send notifications
- Update client code
- Register device and subscribe to notifications
- Update backend code
- Connect to Notification Hub
- Send notifications
1. Create a Mobile App in Azure
Straightforward. Refer Azure docs if required.
2. Add Notification Hub to Mobile App
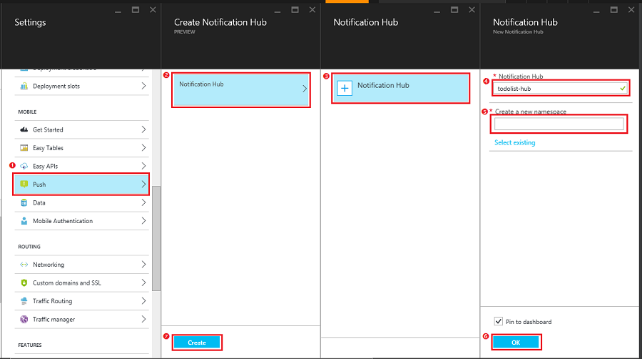
Refer Azure docs if required.
3. Register in PNS
Create an account in the required PNS, and keep note of some keys.
For FCM, create a new project, go to project settings, and under Cloud Messaging tab, keep note of the SENDER_ID and Server Key.
4. Configure back-end to send notifications
Again under push settings of the Mobile App in Azure, choose the PNS (GCM for Android), and add the Server Key noted earlier under API Key. This is to connect the ANH to the PNS, so messages can be sent from the server to the client app via the PNS.
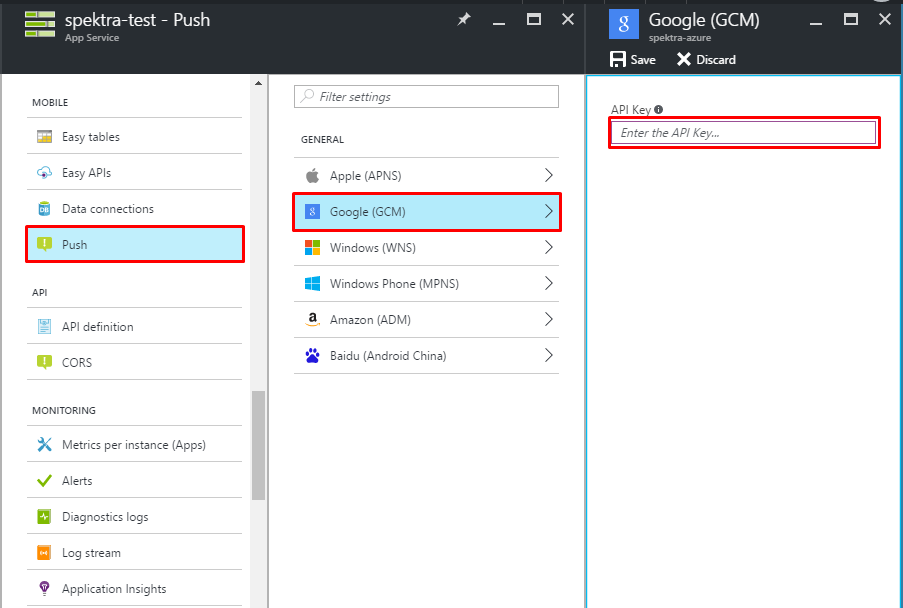
Please note that each PNS defines different methods to connect to them.
5. Update code
In client/device, registering device and subscribing to notifications:
For Ionic/Cordova apps, two plugins should be added:
- cordova-plugin-ms-azure-mobile-apps This is to connect with the Azure Mobile App that was created to handle the backend part of the app.
- phonegap-plugin-push This plugin is now the defacto plugin used for push notifications in cordova.
First, connect app to PNS with phonegap-plugin-push.
Initialising the push notification plugin with the required data to connect to the PNS.
pushRegistration = PushNotification.init({
android: { senderID: 'SENDER_ID' },
ios: { alert: 'true', badge: 'true', sound: 'true' },
wns: {}
});
Registering the app with the PNS:
pushRegistration.on('registration', function (data) { ... }
On successfule registration, a registrationId is obtained from the PNS (in case of Android).
Next, connect the app with the Azure Mobile app, with cordova-plugin-ms-azure-mobile-apps
var client = new WindowsAzure.MobileServiceClient('URL to mobile app');
Initialising.
After registration with PNS, add the app to ANH:
client.push.register('gcm', registrationId, {
mytemplate: { body: { data: { message: "{$(messageParam)}" } } }
});
For GCM, similar for others too. mytemplate defines the template of each push message.
To listen for push messages:
pushRegistration.on('notification', function (data, d2) {
// push message will be in data.message
});
Handling errors:
pushRegistration.on('error', handleErrorCallback);
Backend:
Test push notifications can now be sent to each registered device from ANH. The server code must be updated to send push notifications based on some triggers, which could be calling an API, or adding an entry to a DB table. This part includes connecting to ANH from the mobile app and using the connection established earlier with the PNS to send notification messages.
Refer here for more implementation details.
You can test push messages from the Portal, or from Visual Studio (if connected).
Disclaimer: Implementation details may change.
Issues encountered:
- There was a problem connecting the ANH to FCM, which is unexplained. Adding the Server Key to the GCM API Key in ANH resulted in a ‘Error updating notification hub message’. This got solved after a few hours on its own. UPDATE: This error occurs when CORS is force-enabled in the browser. A lot of Azure functions behave weirdly when CORS is enabled